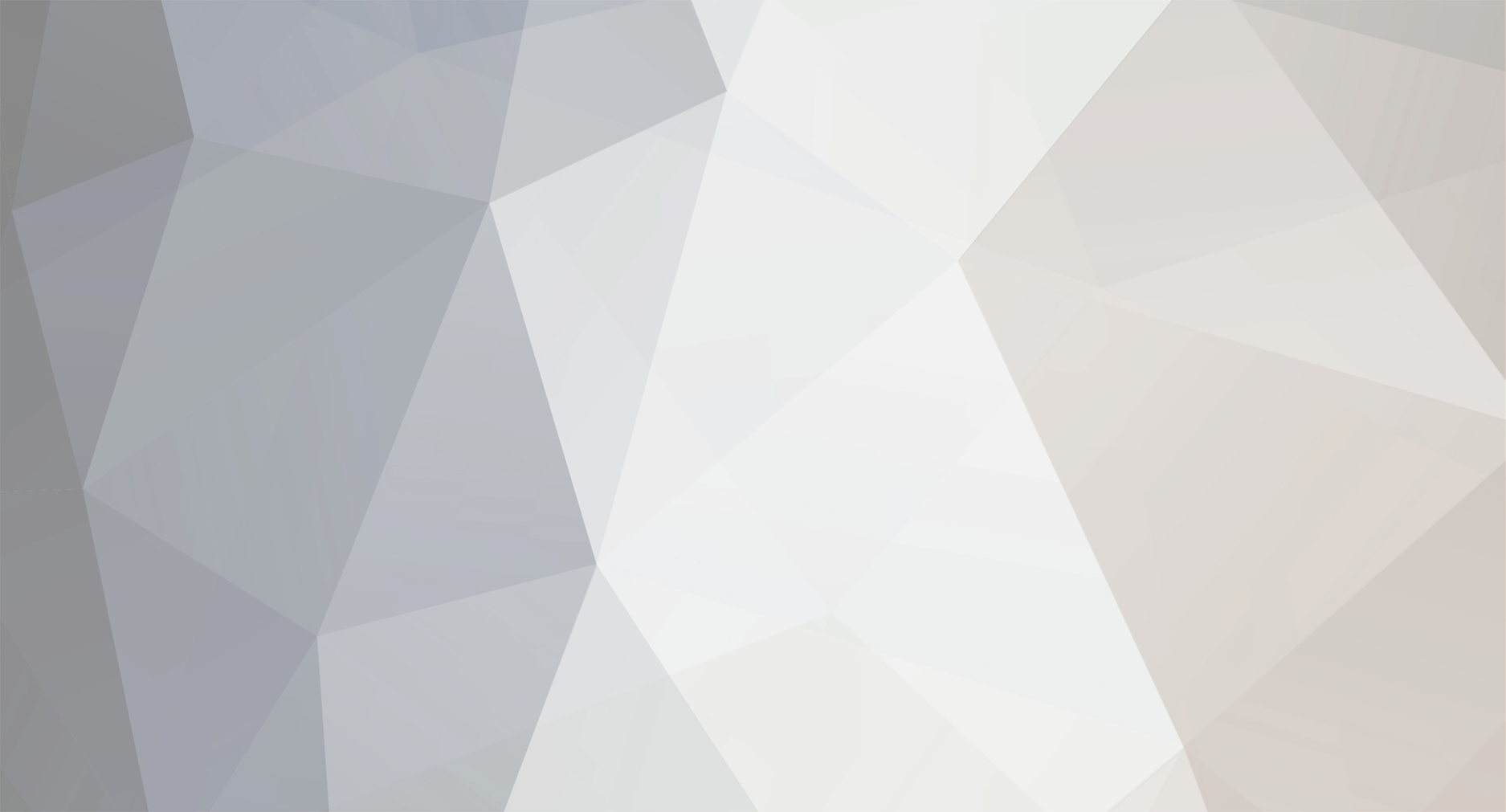
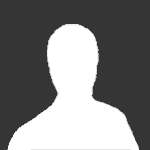
WalidKhan
Members-
Posts
18 -
Joined
-
Last visited
Profile Information
-
Favorite Area of Science
computer
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
WalidKhan's Achievements

Quark (2/13)
0
Reputation
-
Hello there. So I was reading this blog (url deleted) and decided to fall into the field of web development, and I'm feeling a little overwhelmed by all of the options. I want to make a good strategy to guide my study, but I don't know where to begin. Can you help me figure out the best next steps? Here's what I'm thinking: What are the important steps I should follow to get started in web development? Should I learn front-end code, back-end coding, or both? What are the easiest programming languages and tools for newbies like me to learn? Can you recommend any beginner-friendly materials or courses that can help me get started? I'd be super grateful for any guidance or advice you can offer to help me chart my course in web development. Thanks a ton for your support! Best wishes,
-
i am going over the complex process of reading data from files. Let's start by studying a code sample that attempts to read data from a file: # Opening a file for reading file = open("data.txt", "r") data = file.read() print(data) file.close() At first sight, this code appears easy. However, a deeper investigation shows some subtle places for improvement: To begin, error management is an important part of file operations since it ensures resilience and dependability. This code sample lacks error handling tools to deal with probable difficulties like file not found or unavailable. Incorporating error handling logic into try-except blocks can improve code resiliency. Second, the lack of a context manager (with open() as file:) for file opening is notable. Using a context manager provides effective resource management and automated file closure, even in the face of exceptions. This method encourages cleaner and more efficient coding. Third, the code assumes the existence of the file "data.txt" without checking. Adding validation to check for the file's existence before opening it is critical for avoiding mistakes and increasing the code's stability. Finally, supplying the encoding when opening files is advised for appropriate handling of diverse text encodings, as demonstrated Commercial link removed by Moderator. By default, files are opened in text mode, which might cause encoding difficulties when working with non-ASCII characters. Explicitly supplying the encoding argument in the open() method can help alleviate these problems and assure correct data treatment. Can you assist me in identifying and addressing these four areas for improvement in the attached code snippet? Your understanding of file reading in Python will be beneficial to our discussion.
-
Hello Python community! đ. I'm exploring the fascinating world of interpreted and compiled languages, with a particular concentration on Python. Can somebody describe the fundamental distinctions between interpreted and compiled languages, and how Python's simplicity fits into the interpreted landscape? I'd also want to see some hands-on Python examples demonstrating its interpreted nature - any takers? As we negotiate the Python terrain, could someone give insights regarding the benefits and drawbacks of interpreted languages, particularly in the Python development community? What makes Python tick, and where may we stumble on our Pythonic journey? Switching gears to performance, are there any Python experts out there? What optimization techniques and code snippets do you swear by for increasing runtime performance in Python's interpreted realm? Now, let us talk about languages that compile to Python. Do you have any experiences or recommendations? What advantages do they offer, and can you provide real-world instances supported with Pythonic code examples? To top it all off, does anyone have a useful Python code template for hands-on exploration? After reading that post (link removed by mod), I'm eager to experiment with it and obtain a better grasp of the dance between the interpreted and compiled paradigms in Python.
-
I am developing a web application using Node.js and Express.js. However, encounter a challenge with routing that involves choosing between using the core Node.js http module for routing or leveraging Express.js for the same purpose. Consider the following code snippet: const http = require('http'); const url = require('url'); const server = http.createServer((req, res) => { const path = url.parse(req.url).pathname; if (path === '/home') { res.writeHead(200, {'Content-Type': 'text/plain'}); res.end('Welcome to the home page!'); } else if (path === '/about') { res.writeHead(200, {'Content-Type': 'text/plain'}); res.end('Learn more about us!'); } else { res.writeHead(404, {'Content-Type': 'text/plain'}); res.end('Page not found!'); } }); const port = 3000; server.listen(port, () => { console.log(`Server listening on port ${port}`); }); I'm also looking at multiple articles to discover the solution to the issue of identifying the benefits and drawbacks of utilizing this simple Node.js http module technique for routing against Express.js. Furthermore, propose a concise solution to improve the code depending on the chosen routing technique. Thank you for delving into this Express.js vs Node.js programming challenge!
-
Given an array of integers, find the length of the longest increasing subsequence. A subsequence is a sequence that can be derived from another sequence by deleting some or no elements without changing the order of the remaining elements. For example: #include <iostream> #include <vector> int longestIncreasingSubsequence(const std::vector<int>& nums) { // Your dynamic programming solution goes here. // Return the length of the longest increasing subsequence. } int main() { std::vector<int> sequence = {10, 22, 9, 33, 21, 50, 41, 60, 80}; int result = longestIncreasingSubsequence(sequence); std::cout << "Length of the longest increasing subsequence: " << result << std::endl; return 0; } I'm specifically interested in implementing this using dynamic programming techniques. How can I approach this problem using dynamic programming, and what would be the C++ code for solving it? Any insights, code snippets, or explanations would be incredibly helpful in mastering dynamic programming for this particular challenge. Thank you for your assistance!
-
I'm using an online Java compiler for my project, and I'm encountering unexpected behavior in the execution of my code. Here's a simplified snippet: import java.util.ArrayList; public class Main { public static void main(String[] args) { ArrayList<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); for (String fruit : fruits) { System.out.println(fruit.length()); } } } Despite the seemingly straightforward code, I'm not getting the expected output. What could be causing this issue with the online Java compiler, and how can I rectify it to ensure proper code execution and output?
-
DNA computers are a theoretical concept that use DNA molecules to perform computations. They work by encoding data in DNA sequences and manipulating them through biochemical processes. The key steps are: Data Encoding: Information is stored as sequences of DNA molecules. DNA's four base pairs (A, T, C, G) represent binary data (0 and 1). Operations: Biochemical reactions, such as polymerase chain reactions (PCR) and DNA hybridization, are used to manipulate and process the DNA strands. Parallelism: DNA computers can perform massively parallel computations by processing many DNA strands simultaneously. Readout: The final DNA sequence is decoded to extract the computed result. While the concept is intriguing, DNA computers are still in the experimental and theoretical stage and face many challenges, including error rates and practical implementation issues. They are not yet used in practical computing.
-
I'm learning C++ and trying to grasp control structures. I have an array of integers and I want to iterate through it using different control structures to achieve the same result. Here's my array: int numbers[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; I would like to calculate the sum of all the elements in the array and display the result. Can you provide code examples using different control structures like for loops, while loops, and do...while loops to accomplish this task? Additionally, if there are any performance or readability differences between these approaches, please explain. I have looked online for a solution, but I want to know how to use control structures in C++ successfully in a variety of scenarios.
-
I'm working on a data analysis project in Python and need to calculate both the mean and median of a dataset. I understand the basic concepts, but I'm looking for a Python code example that demonstrates how to do this efficiently. Let's say I have a list of numbers: data = [12, 45, 67, 23, 41, 89, 34, 54, 21] I want to calculate both the mean and median of these numbers. Could you provide a Python code snippet that accomplishes this? Additionally, it would be helpful if you could explain any libraries or functions used in the code. Thank you for your assistance in calculating these basic statistics for my data analysis project!
-
In a web development project, I'm dealing with two lists of data that need to be merged and displayed on a webpage. One list contains user comments, and the other list contains system-generated messages. I need to merge these two lists while preserving the order of messages and displaying them on the webpage. Here's a simplified example of the data: user_comments = [ {'user': 'Alice', 'message': 'Hello!'}, {'user': 'Bob', 'message': 'Hi there!'}, ] system_messages = [ {'message': 'System message 1'}, {'message': 'System message 2'}, ] I want to merge these two lists to create a single list that combines user comments and system messages in the correct order for display on the webpage. What considerations should I make when combining and presenting these lists on a webpage as part of a web development project, and how can I accomplish this in Python? I attempted to locate the answer by visiting different sites, but I was unable. Could you give an example of code and describe how to format the combined data? Thank you for your guidance!
-
I'm seeking assistance in exporting data from a Django model to a CSV file using the csv module in Python. I've read about the process, but I'm struggling to put together the necessary code within my Django project. Could someone kindly provide a code example or guide me through the process? Here's the simplified version of my Django model: # models.py from django.db import models class Product(models.Model): ProductID = models.IntegerField() ProductName = models.CharField(max_length=100) Price = models.DecimalField(max_digits=10, decimal_places=2) I want to export the data from the Product model to a CSV file named products.csv. How can I achieve this using the csv module along with Django's ORM? I'd greatly appreciate it if someone could provide a code snippet or step-by-step explanation to help me get this CSV export functionality up and running within my Django project. Thank you for your assistance!
-
I am currently working on implementing the Quick Sort algorithm in Python, and while my code seems to work correctly, I am eager to optimize it for better performance and adhere to best practices. I would appreciate insights from the community on how to fine-tune my Quick Sort implementation to make it more efficient, especially when dealing with large datasets. Are there specific techniques, pivot selection strategies, or partitioning schemes that are recommended for faster sorting? Additionally, I would like to know how to handle edge cases and prevent potential issues like stack overflow during recursion, especially when dealing with arrays that are nearly sorted or already sorted. Here's my current implementation: import random def quick_sort(arr): _quick_sort(arr, 0, len(arr) - 1) def _quick_sort(arr, low, high): if low < high: # Randomly select the pivot to avoid worst-case scenarios pivot_index = random.randint(low, high) arr[high], arr[pivot_index] = arr[pivot_index], arr[high] # Perform the partition and get the pivot index pivot_index = partition(arr, low, high) # Recursively sort the two partitions _quick_sort(arr, low, pivot_index - 1) _quick_sort(arr, pivot_index + 1, high) def partition(arr, low, high): pivot = arr[high] i = low - 1 for j in range(low, high): if arr[j] <= pivot: i += 1 arr[i], arr[j] = arr[j], arr[i] arr[i + 1], arr[high] = arr[high], arr[i + 1] return i + 1 # Sample usage: unsorted_array = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5] quick_sort(unsorted_array) print(unsorted_array) In this implementation, the _quick_sort function handles the recursive sorting, while the partition function rearranges the elements around the pivot using the Lomuto partition scheme. We use the random pivot selection strategy to avoid worst-case scenarios and improve average performance. It is worth mentioning that even with these optimizations, Quick Sort's worst-case time complexity is O(n^2) for an already sorted or nearly sorted array. However, the random pivot selection helps to mitigate this issue in practice, making Quick Sort generally efficient and widely used in many sorting applications. For my initial understanding of the subject, I have been using articles on Scaler's Quick Sort Algorithm, but I'm open to suggestions and code samples that show how to optimize the Quick Sort algorithm in Python while maintaining its correctness and making sure it operates robustly in a variety of scenarios. Thank you for your valuable expertise and time!
-
I'm attempting to understand 'self' in Python on a deeper level since I find myself not fully comprehending it when I use it. Consider the following code: class FoodItems: def __init__(self, other arguments): some code def main(): some code item1 = FoodItems item1.main() For the [def main()] function I'm not passing the 'Self' argument in it, yet the code works when I call it. After reading this post, my understanding of 'Self' is that it must be provided every time a method is defined under the class. I receive the error (missing 1 needed positional argument:'self') if I pass 'Self' and then call the method. Could someone please clarify this to me? When it comes to generating instances, I can't discern the difference between calling an instance with or without brackets, such as (item1 = FoodItems vs. item1=FoodItems()). When I use parentheses, I receive the error "missing 8 required positional arguments that were initialized in the constructor." Any assistance would be highly appreciated; thank you in advance!
-
I'm attempting to compile a hello world c programme on the mac terminal and getting the following errors: mysource.c:1:19: error: /usr/local/include/stdio.h: Permission denied mysource.c: In function âmainâ: mysource.c:2: warning: incompatible implicit declaration of built-in function âprintfâ This is what I typed into the terminal (the name of the.c file is mysource): MacBook-Pro:~ drummer014$ gcc mysource.c -o mysource I had xcode 3 installed and received the same error, then I installed xcode 4.2 today and am experiencing the same issue. I'm using a MacBook Pro running Snow Leopard. I have tried typing gcc and then dragging the file into the terminal after following this scaler guide, but I still get the same issue. Any assistance would be highly appreciated. I used the command gcc âverbose mysource.c -o mysource. What I got was as follows: Using built-in specs. Target: i686-apple-darwin10 Configured with: /private/var/tmp/llvmgcc42/llvmgcc42-2336.1~3/src/configure --disable- checking --enable-werror --prefix=/Developer/usr/llvm-gcc-4.2 --mandir=/share/man --enable-languages=c,objc,c++,obj-c++ --program-prefix=llvm- --program-transform-name=/^[cg][^.-]*$/s/$/-4.2/ --with-slibdir=/usr/lib --build=i686-apple-darwin10 --enable- llvm=/private/var/tmp/llvmgcc42/llvmgcc42-2336.1~3/dst-llvmCore/Developer/usr/local --program-prefix=i686-apple-darwin10- --host=x86_64-apple-darwin10 --target=i686-apple-darwin10 --with-gxx-include-dir=/usr/include/c++/4.2.1 Thread model: posix gcc version 4.2.1 (Based on Apple Inc. build 5658) (LLVM build 2336.1.00) /usr/llvm-gcc-.2/bin/../libexec/gcc/i686-apple-darwin10/4.2.1/cc1 -quiet -v -imultilib x86_64 -iprefix /usr/llvm-gcc-4.2/bin/../lib/gcc/i686-apple-darwin10/4.2.1/ -D__DYNAMIC__ mysource.c -fPIC -quiet -dumpbase mysource.c -mmacosx-version-min=10.6.8 -m64 -mtune=core2 -auxbase mysource -version -o /var/folders/7r/7rMZhHx3F0WhnoyEK1zUgE+++TI/-Tmp-//ccaqeJkd.s ignoring nonexistent directory "/usr/llvm-gcc-4.2/bin/../lib/gcc/i686-apple-darwin10/4.2.1/../../../../i686-apple-darwin10/include" ignoring nonexistent directory "/Developer/usr/llvm-gcc-4.2/lib/gcc/i686-apple-darwin10/4.2.1/../../../../i686-apple-darwin10/include" #include "..." search starts here: #include <...> search starts here: /usr/llvm-gcc-4.2/bin/../lib/gcc/i686-apple-darwin10/4.2.1/include /usr/local/include /Developer/usr/llvm-gcc-4.2/lib/gcc/i686-apple-darwin10/4.2.1/include /usr/include /System/Library/Frameworks (framework directory) /Library/Frameworks (framework directory) End of search list. GNU C version 4.2.1 (Based on Apple Inc. build 5658) (LLVM build 2336.1.00) (i686-apple- darwin10) compiled by GNU C version 4.2.1 (Based on Apple Inc. build 5658) (LLVM build 2336.1.00). GGC heuristics: --param ggc-min-expand=150 --param ggc-min-heapsize=131072 Compiler executable checksum: e787fa4ffdc9e78ad5e913828c220d85 mysource.c:1:19: error: /usr/local/include/stdio.h: Permission denied mysource.c: In function âmainâ: mysource.c:3: warning: incompatible implicit declaration of built-in function âprintfâ
-
I'm newbie to algorithms and also was working upon developing a Quick Sort algorithm with such a duplex partition to ensure that it works quickly even on sequences with many equal components. My approach was as follows: def randomized_quick_sort(a, l, r): if l >= r: return k = random.randint(l, r) a[l], a[k] = a[k], a[l] #use partition3 m1,m2 = partition3(a, l, r) randomized_quick_sort(a, l, m1 - 1); randomized_quick_sort(a, m2 + 1, r); def partition3(a, l, r): x, j, t = a[l], l, r for i in range(l + 1, t+1): if a[i] < x: j += 1 a[i], a[j] = a[j], a[i] elif a[i]>x: a[i],a[t]=a[t],a[i] i-=1 t-=1 a[l], a[j] = a[j], a[l] return j,t It doesn't provide properly sorted lists. after following through this article I discovered the right partition code implementation. def partition3(a, l, r): x, j, t = a[l], l, r i = j while i <= t : if a[i] < x: a[j], a[i] = a[i], a[j] j += 1 elif a[i] > x: a[t], a[i] = a[i], a[t] t -= 1 i -= 1 # remain in the same i in this case i += 1 return j,t Could you please clarify why the wrong partition implementation failed? Thank you in advance.